这里记录了Java文件IO和控制台IO的使用方法。
IO分为两种:字节流和字符流。字节流就是每次读取以字节为单位。字符流就是每次读取都以字符为单位。
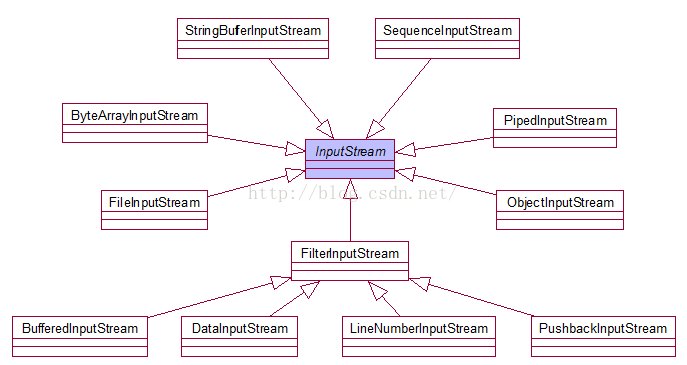
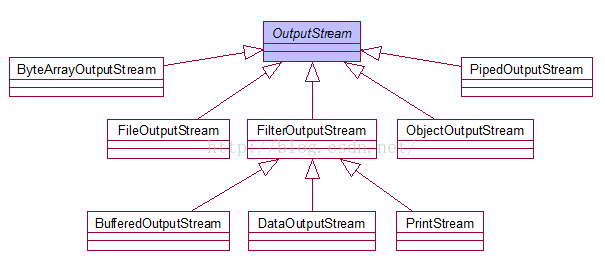
所有的字节流IO的父类都是InputStream
和OutputStream
。
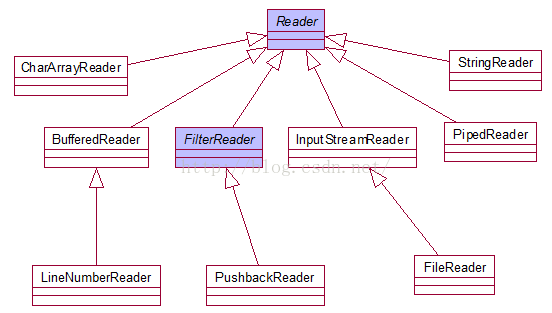
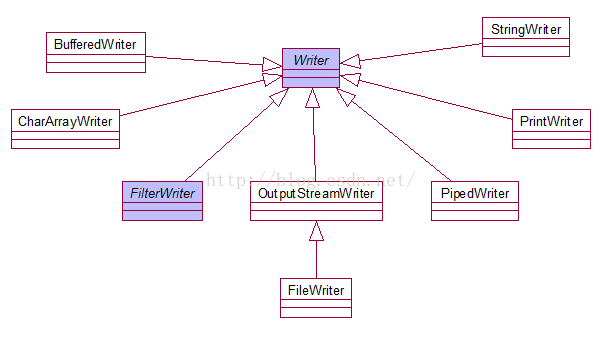
所有的字符流的IO的基类全都是Reader
和Writer
。
对于InputStream
,其最重要的接口方法就是read()
,那么我们可以这样写:
1
2
3
4
5
6
7
8
9
|
InputStream stream = new DataInputStream(System.in);
int a = 0;
try {
while ((a = stream.read()) != -1) {
System.out.print((char) a);
}
} catch (IOException e) {
e.printStackTrace();
}
|
read()
方法会返回当前读取的数据长度(0~255),没有数据返回-1.
输出的话我们有System.out.print()
系列函数。
首先我们需要有一个描述文件的类,就像C/C++文件句柄一样。File
类就是这样一个类。可以给它一个路径来创建一个文件句柄:
1
|
File file = new File("~/Documents/program");
|
但是这个类不会自己判断文件或者文件夹是否存在,你可以使用exists()
函数判断。它还有很多其他实用的函数,比如getAbsolutePath()
得到给定的路径的绝对路径,或者调用createNewFile()
或者mkdir()
来创建位于此路径的文件和文件夹。
有了File类,我们再对照上面的图,可以看到我们可以使用FileInputStream
类对文件读,FileOutputStream
对文件写:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
File file = new File("./test.txt");
if (!file.exists())
try {
file.createNewFile();
} catch (Exception e) {
e.printStackTrace();
}
try {
OutputStream ostream = new FileOutputStream(file);
char[] b = { 'h', 'e', 'l', 'l', 'o' };
for (char c : b)
ostream.write((int) c);
} catch (Exception e) {
e.printStackTrace();
}
try {
InputStream istream = new FileInputStream(file);
int i = 0;
while ((i = istream.read()) != -1)
System.out.println((char) i);
} catch (Exception e) {
e.printStackTrace();
}
}
|
这里先写文件再读文件。
或者你也可以使用字节流,用法都一样,只不过换一个类:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
File file = new File("./test.txt");
if (!file.exists())
try {
file.createNewFile();
} catch (Exception e) {
e.printStackTrace();
}
try {
OutputStreamWriter ostream = new FileWriter(file);
ostream.write("hello");
ostream.flush(); //这里使用flush表示立刻写入,不然可能再下面读取的时候还没有写入。
} catch (Exception e) {
e.printStackTrace();
}
try {
InputStreamReader istream = new FileReader(file);
char[] buffer = new char[10];
istream.read(buffer, 0, 10);
System.out.println(buffer);
} catch (Exception e) {
e.printStackTrace();
}
|